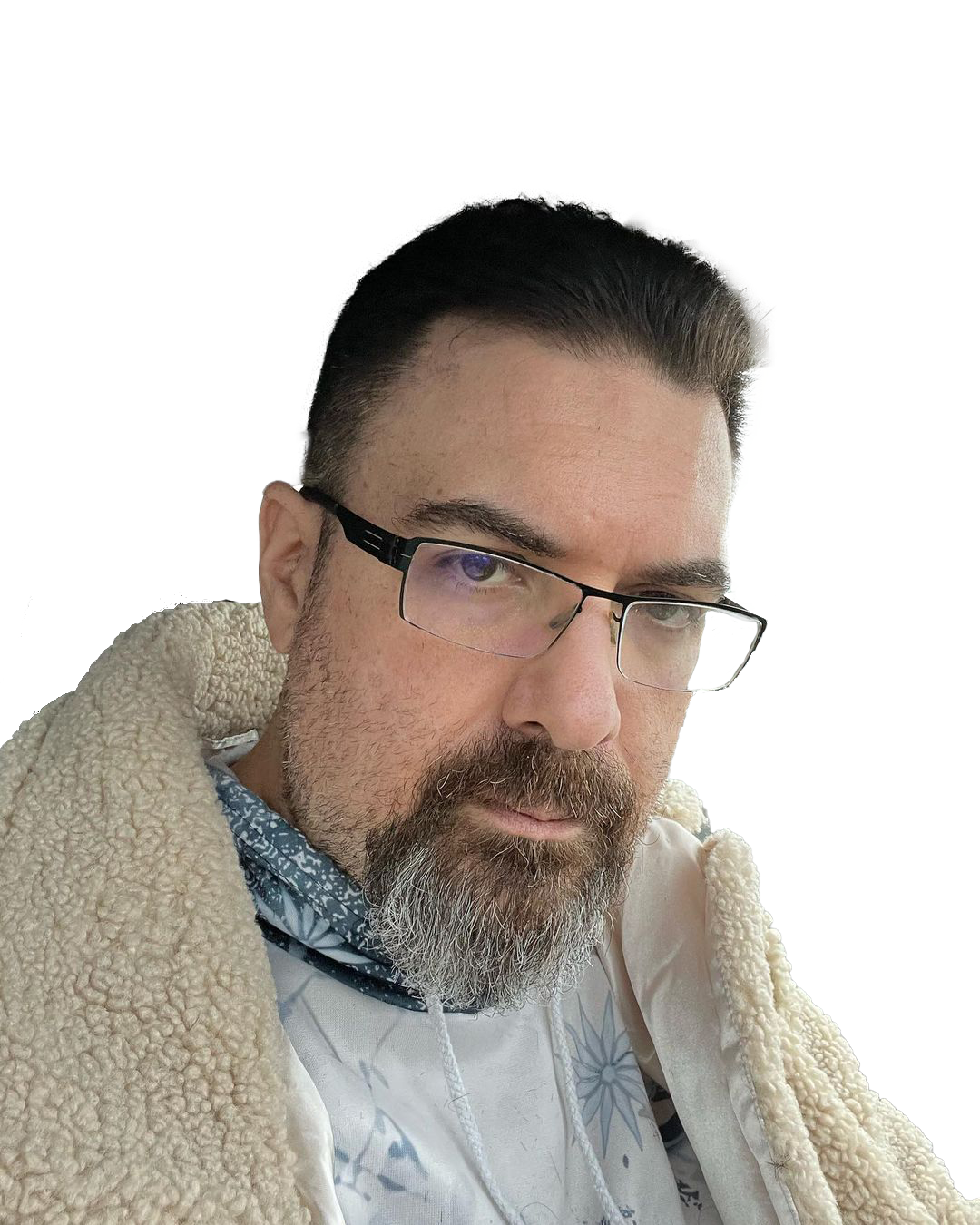
bind
by JasonML
There are those times I come across an article that are so amazingly simple and obvious that it immediately changes the way I write code. Understanding JavaScript's Function.prototype.bind is one such article. It's one of those articles that talks about something I always knew but never really thought about it that way before.
I'll let the article speak for itself, but I want to give you the pattern I enjoy now. I want to show you how it makes itself easy to approach and use.
So, let's say we have this bit of code.
Buckets.prototype.add = function(data){ var stopEarly = ! (this.options.stop_on_match === true); _.each(this.bucketConditions, function(c){ if(c.test(data)){ this.buckets[c.name].push(data); return stopEarly; } }.bind(this)); return this;};
This is part of a buckets node package I'm finishing up. Now, I want 'data' to be whatever they pass in, so this could be an object, an array, or whatever. Because of that, however, I need to create another function that allows the user to enter a data list.
Buckets.prototype.addList = function(data, cb) {...};
I want to reuse the 'add' function already created. So I add this:
Buckets.prototype.addList = function(data, cb){ var that = this; async.each(data, function(v){ that.add(v); });};
We've all seen code like that. However, the article mentioned above reminds you about .bind, and its special ability to bind the function to a certain object as context. This means instead of writing the above code, I can do this:
Buckets.prototype.addList = function(data, cb){ async.each(data, this.add.bind(this), cb);};
This is so much easier, and so much more elegant. I highly suggest reading the above article. It's wonderful.