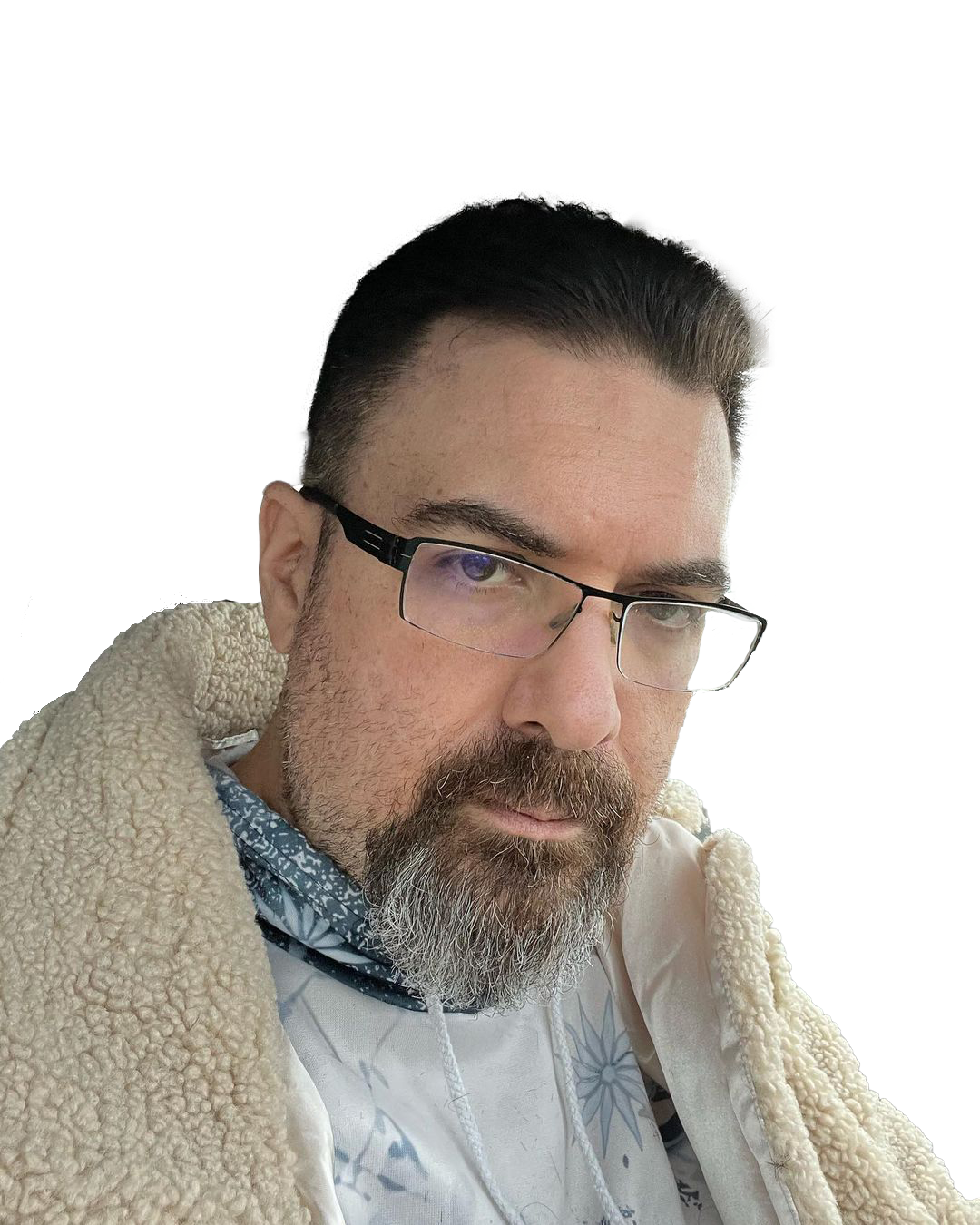
PHP Tips and Tricks: Array Lengths
by JasonML
This is a simple trick. It makes the assumption that you manage your arrays properly, and in this case, you are using an array (or list), and not a dictionary. Basically, a list that starts at 0 (though, the method can be adjust to fit other starting points).
Anyways, there are times I need to know if an array contains 15 elements. I can quickly discover this like so:
if ( isset($array[14]) ) {
All this does is check to see if there is a value set for element 14. Again, this makes the assumption that the interface you are calling returns what it says it will. It should, and if it doesn't, that's a serious bug.
This also comes in handy if I only want to return a subset of the information, and need to find out if there is more information. Let's say I want to return as little information as possible via the API (I only want to return the first page of results), but I also want to know if there are more results (for pagination, let's say). I can then ask the API for 16 results, and then check if there are more results like so:
$isMore = isset($array[15]);
With this simple technique, I need only return 16 results. I don't need to do anything else fancy, just return 16 results, display 15 of them, and recognize that there are more results.
Finally, you can use this technique to ensure the length of a string. So, lets say we want to enforce a minimum line length for passwords, we can enforce it like so.
$isPasswordLongEnough = isset($password[7]);
Obviously in all these cases, we have to be aware of off-by-one errors. For example, $password[7] checks for a password of at least 8 characters, not 7.